Introduced in WordPress 2.5, the shortcode API has given developers a handy way of including dynamic content within the CMS or individual theme files. In this WordPress shortcode tutorial, I’m going to show you how to register and use your very own shortcode.
Shortcodes can be used to pull a variety of functions and dynamic content from a form to your latest post. The simplest usage of shortcode looks a little like this:
[jamesshortcode]
1. Prepare a function to call out when the shortcode is used
For this tutorial, we’re going to register a shortcode that will call and execute a function. For this example we’ll create a simple function that will tell us who and when the page/post was last modified. The contents of this function would look a little like this:
<?php return "<p>This post was last edited on ".get_the_modified_date()." by ".get_the_modified_author()."</p>"; ?>
Ordinarily, if we wanted to include and deploy this code within a post’s CMS, we wouldn’t be able to (without the assistance of a plugin or shortcode). However if we register a Shortcode, we can swap the shortcode and deploy our function.
2. Create your Callback Function
Once found, registered shortcodes are swapped for your code, which is known as the Callback Function. So first of all, we need to create our Callback Function and register our new shortcode. Simply open your theme’s functions.php file and create an empty function with an appropriate name. I’ve called my Callback Function ‘last_updated_code’:
function last_updated_code() { }
Now that you’ve created and named your function, simply return your code or included PHP file inside the curly brackets like this:
function last_updated_code() { return "<p>This post was last edited on ".get_the_modified_date()." by ".get_the_modified_author()."</p>"; }
It’s important to ‘return’ your code rather than ‘include’ or ‘echo’ which will not work.
3. Register your shortcode
The last thing we need to do is call the API and register our shortcode. The shortcode handler has two parameters. The first is the name of our shortcode (the actual shortcode we’ll use in the CMS) and the second is the name of the function we’ve already created:
add_shortcode( 'lastupdated', 'last_updated_code' );
Make sure that your new shortcode name is unique to avoid clashing with common plugin shortcodes.
4. Putting it altogether
Here’s the complete code which I’ve stored as a Gist on Github:
And below this sentence is the actual output from my shortcode that I’ve used as an example in this tutorial:
This post was last edited on September 25, 2013 by
5. Using the shortcode
The most common place you’ll want to use your shortcode is within the WYSIWYG edit on pages or posts. Here’s a screenshot I took whilst editing this page, so you can see how the example above was rendered:
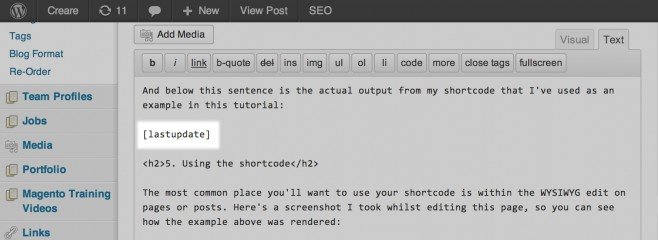
If however, you wish to use the Shortcode within your theme’s template files you simply need to wrap it inside the WordPress tag ‘do_shortcode()’ like this:
<?php echo do_shortcode("[lastupdated]"); ?>
However Konstantin Kovshenin does make a good point about whether you actually should use shortcodes within your template files when you can just call the function directly.
In a follow up post I’m planning, I’m going to build on this tutorial by introducing attributes to the shortcode.