In this tutorial I’m going to show you a few examples of broken “Latest Tweets” implementations across a number of different websites, why these are now broken and also a good method of fixing them using Twitteroauth.
In a follow-up post I’m going to show you how you can use the Twitter Search API 1.1 to create a nice piece of functionality similar to the latest tweet section on our own website using the Magento infrastructure!
I’ve been browsing the web and I can see plenty of examples of where this latest tweet functionality has really fallen on it’s face – but it’s not entirely the developers fault. Twitter have recently closed down their old API (version 1.0) and have instead forced users to connect via their Oauth enabled API 1.1. This means that all implementations of the old API version will now simply stop receiving information, leaving large blank areas across many well-known websites.
You may be used to seeing things like this at the moment:
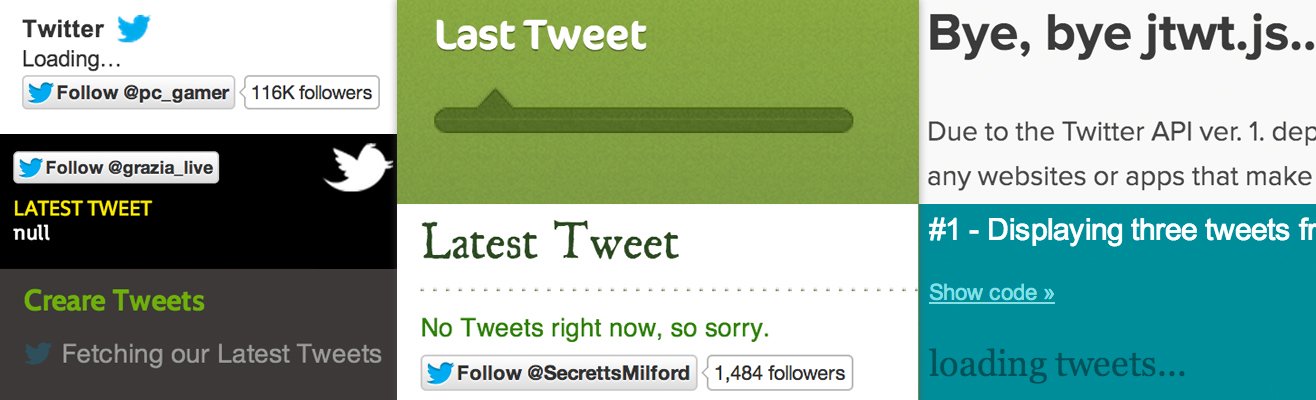
As you can see – we also fell victim to this API change (bottom left).
jQuery & Javascript Latest Tweets
One major thing to consider is that because Twitter API 1.1 uses Oauth explicitly, as far as I know there are no longer any custom-made Javascript or jQuery based latest-tweet tools out there that still work. The only ones that continue working are Twitter’s own widgets:
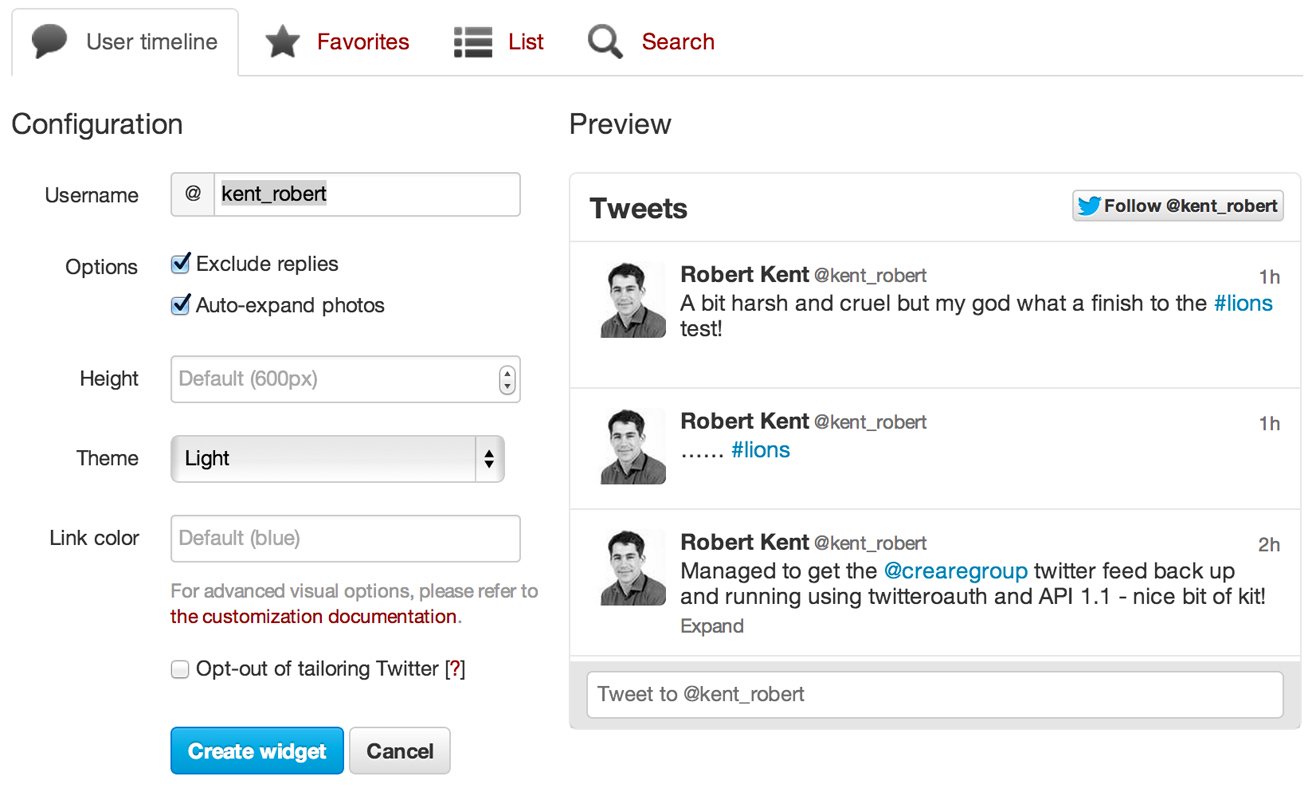
As you can see from the image above – Twitter also allows you to tailor this to your own design quite easily – so this is a really good method of ensuring that your latest tweets display and (more importantly) stay visible whatever twitter decides to do with its code.
PHP Latest Tweets
The main change as far as server-side programming and the API is concerned, is that it’s now only possible to fetch tweets via PHP (using methods such as CURL) only after a successful Oauth verification. This Oauth verification is, at its most basic level, a login. Without logging in (ensuring that you are allowed access to this data) you will be repelled by the API and sent packing.
There are a variety of ways to get around this but by far the easiest is to use the Twitteroauth library and a simple piece of PHP script. These, in conjunction with a registered twitter application, will give you all the access you need to be able to tweet remotely, grab yours or others latest tweets and also perform twitter searches using the Twitter Search API.
To make this into a tutorial here are a few really simple steps to solve the PHP Latest Tweet problem – bear in mind that this is basic code – you should think carefully about integrating this into your own code / framework.
Step 1 – Create Twitter Application
The first step is to register our twitter application. This is a must! It will give us all the secret information that we need in order to “login” to the Twitter API.
Visit https://dev.twitter.com and login with your twitter credentials. Once logged in you should be able to click on your image top-right and choose My applications.
Next, click on the “Create New Application” button, here you need to enter your name, description and website URL. Once complete you should be presented with a page like this:
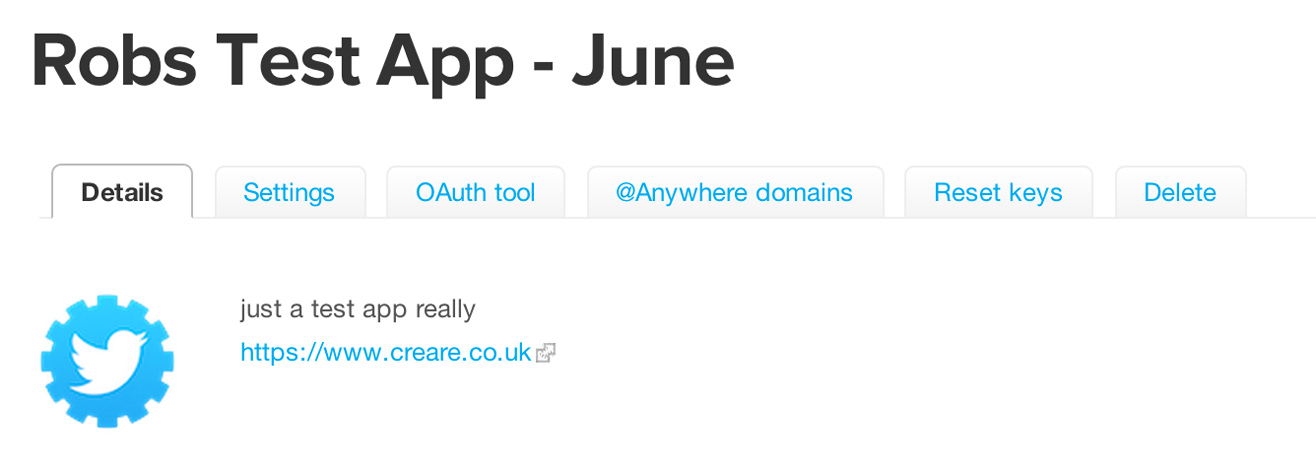
On this page you will find your consumer key and consumer secret key. If you want to be able to post to twitter then you may want to change your Access level from Read-Only to Read and Write (and DM if you like) by clicking on the settings page.
The last thing to do here is to create the token, scroll to the bottom of the Details page and click Create my Access Token, if you change your access level after this point you will need to re-create this token.
Now that you’ve created your token you should have the following bits of information:
- Consumer Key
- Consumer Secret Key
- Access Token
- Access Token Secret
Believe it or not, that’s all you need to adjust in the following code to get your new latest tweets to work. For those who still struggle after this point – try adding your domain into the @anywhere tab.
Step 2 – Download Twitteroauth and run some code
Now you need to download twitteroauth. I played with a few other libraries for quite some time when the Twitter API change was made but none were so quick and simple as twitteroauth. Once downloaded you need to place the main twitteroauth folder somewhere you can reference in your code – for example on the root layer of your website. Twitteroauth comes with an Oauth library – so you do not need a separate one for this.
Now that Twitteroauth is downloaded and accessible in your code we just need to write something like the following:
require_once($_SERVER['DOCUMENT_ROOT'].'/twitteroauth/twitteroauth.php'); // grab twitteroauth from correct place $screen_name = "kent_robert"; // my twitter username $number_of_tweets = 3; // how many do I want? $consumerkey = "YOUR_CONSUMER_KEY"; $consumersecret = "YOUR_CONSUMER_SECRET"; $accesstoken = "YOUR_ACCESS_TOKEN"; $accesstokensecret = "YOUR_ACCESS_TOKEN_SECRET"; $twitterconn = new TwitterOAuth($consumerkey, $consumersecret, $accesstoken, $accesstokensecret); $latesttweets = $twitterconn->get("https://api.twitter.com/1.1/statuses/user_timeline.json?screen_name=".$screen_name."&count=".$number_of_tweets); /* echo '<pre>'; var_dump($latesttweets); // if we want we can see all our data - to check what's available to us in the loop below echo '</pre>'; */ foreach($latesttweets as $tweet){ echo 'Tweet: '.$tweet->text.'<br/>'; // echo my tweet echo 'Tweeted On: '.date('H:i:s d/m/Y',strtotime($tweet->created_at)).'<br/>'; // echo when it was tweeted (nicely) echo 'By: '.$tweet->user->screen_name.'<br/>'; // echo my name }
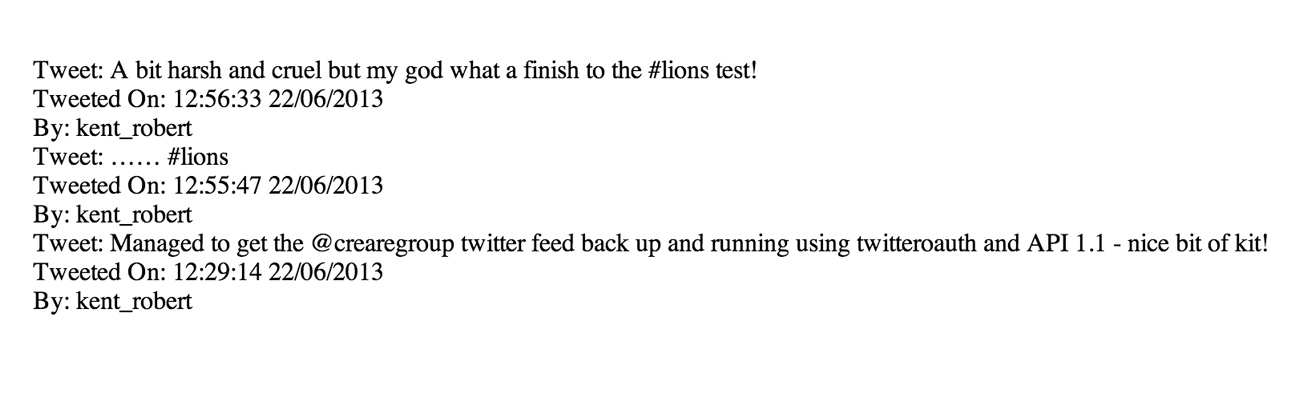
It really is as simple as that! There are lots of different things that you can get from the results – so check out the var_dump in the example above. You can also send Twitter different parameters – so check out their twitter api 1.1 documentation.
Any questions please leave them below.
If you’re interested in our Magento and WordPress integrations have a look at our Advanced Twitter Feed Integration plugin for WordPress and our Creare Twitter extension for Magento.